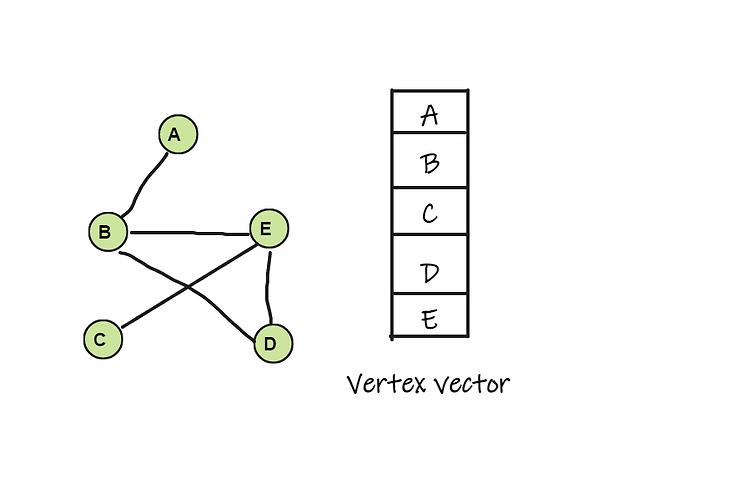
[Algorithm, JavaScript] 그래프(graph)
2022. 10. 25. 12:21
개발 기초/Data Structure & Algorithm
그래프 개요 그래프는 버텍스와 아크로 이루어져 있다. 그래프는 버텍스 간에 여러 개의 아크가 존재할 수 있는데, 다른 버텍스에서부터 오는 아크의 개수를 In-degree, 다른 버텍스로 가는 아크의 개수를 Out-degree라고 부른다. 이때 방향성을 띄고 있는지에 따라 방향 그래프와 무방향 그래프로도 나뉜다. 뱡향 그래프는 무방향 그래프와 다르게 방향을 나타내는 화살표가 있다. 위 방향 그래프 그림에서 (C) 버택스는 (B)로 부터 오는 한 개의 In-degree와, (E), (D)로 가는 두 개의 Out-degree를 가지고 있다. 위 무방향 그래프 그림에서 (E) 버텍스는 (B), (D) 2개의 Degree가 있다. 그래프를 코드로 나타내기 그래프를 코드로 표현하는 방법엔 크게 두 가지가 있다. 하..
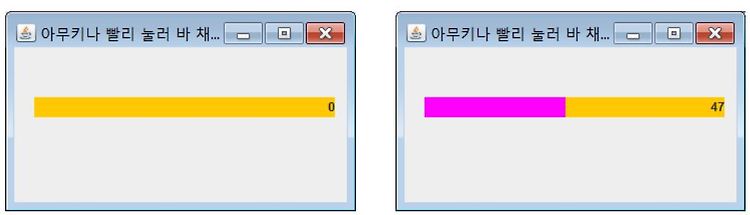
[Java] 생산자-소비자 문제 : wait(), notify()를 이용한 바 채우기
2021. 12. 9. 18:29
Language/JAVA
아무거나 빨리 눌러 바 채우기 import javax.swing.*; import javax.swing.GroupLayout.Alignment; import java.awt.*; import java.awt.event.*; class MyLabel extends JLabel{ int barSize = 0; //바의 크기 int maxBarSize; MyLabel(int maxBarSize){ this.maxBarSize = maxBarSize; } public void paintComponent(Graphics g) { super.paintComponent(g); g.setColor(Color.MAGENTA); int width = (int)(((double)(this.getWidth())) /maxBa..
[JAVA] 파일출력 속도 비교
2021. 11. 19. 19:03
Language/JAVA
import java.io.BufferedOutputStream; import java.io.FileOutputStream; import java.io.IOException; public class WritingPerformanceTest { // 버퍼스트림을 사용해서 성능향상 테스트 public static void main(String[] args) { long start, end; final int FILESIZE = 1000*1000; // 약 1MB final int ARRAYSIZE = 10000; try { FileOutputStream fout = new FileOutputStream("tempfile"); start = System.currentTimeMillis(); //현재 시간을 밀..
[Java] File 클래스를 이용해 ls 명령어 출력해보기
2021. 11. 18. 17:58
Language/JAVA
import java.io.File; import java.util.Date; /* * File 클래스를 이용하여 현재 디렉토리(. )에 있는 파일들에 대해 아래와 *같이 정보를 출력한다. * * . is a directory -RW- 1740 Sat Nov 04 20:01:23 KST 2017 FileClassEx.class -RW- 572 Sat Nov 04 19:03:11 KST 2017 Employee.class DRWX 810 Sat Nov 04 20:01:20 KST 2017 examples … */ public class Myls { public static void main(String[] args) { // TODO Auto-generated method stub File f = new ..
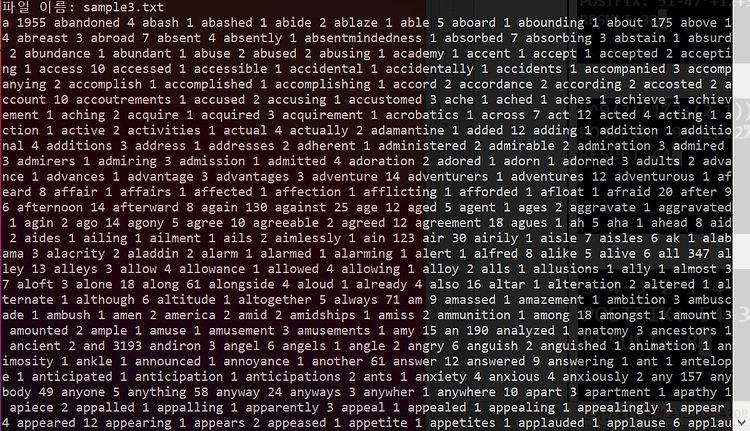
[C/C++, Data Structure] Binary Search Tree를 이용한 단어 빈도 프로그램
2021. 11. 12. 03:10
개발 기초/Data Structure & Algorithm
#include #include #include #include #include #include #define MAX_FNAME 100 #define MAX_WORDS 10000 #define MAX_WORD_SIZE 100 #define TRUE 1 #define FALSE 0 // 데이터 형식 typedef struct { char word[MAX_WORD_SIZE]; // 단어 int count; } element; // 노드의 구조 typedef struct TreeNode { element key; struct TreeNode *left, *right; } TreeNode; // 문자열 비교 strcmp 함수 // 만약 e1 음수 반환 (시스템에 따라: -1) // 만약 e1 ==..
[C/C++, Data Structure] Stack을 사용한 계산기 (infix to postfix)
2021. 10. 26. 04:11
개발 기초/Data Structure & Algorithm
#include #include #include #include // // Calculator // // 프로그램 5.3 // 스택 동작 관련 함수들 typedef char element; #define MAX_STACK_SIZE 100 #define MAX_STR_SIZE 4096 typedef struct { element data[MAX_STACK_SIZE]; int top; } StackType; // 스택 초기화 함수 void init_stack(StackType* s) { s->top = -1; //s->capacity = 1; //s->data = (element *) malloc(s->capacity * sizeof(element)); } // 공백 상태 검출 함수 int is_empty(..